Today I'm going to talk about prototyping custom controls (like buttons) in JSTalk. Why JSTalk? Because it looks like Objective-C, and you're using AppKit classes, and it's super easy to tweak and redraw.
Let's say you're browsing the web, and you come across some fancy web 2.0 button, and you say to yourself "Hey- I wonder what the code would look like for that if I used NSBezier paths?". Well thanks to a new JSTalk plugin I recently wrote, "Image Tools", you can code up your drawing routines in JSTalk and get something like this:
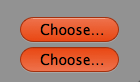
Tweak a single variable, and change it to this:
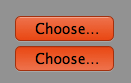
Here's how to get the plugin. First, grab a recent build of JSTalk, even if you have it installed already. Open up the archive, open up the plugins folder, and drag and drop the "ImageTools.jstplugin" file onto JSTalk Editor. This will then launch the editor, and prompt you to install the plugin. Go ahead and do so (this is the new bits, just added today).
Next, download this sample code: nsimage.jstalk. Open it up in JSTalk, and run it. You should get a window with two fake buttons, just like in the images above. Tweak it, and rerun. It's a lot faster than making a new project, adding classes, building, running, etc.
Now let's take a closer look at the code. If you're already a Cocoa programmer, there are a few things to point out:
+[NSImage imageWithSize:] - this is a category that is loaded as part of the Image Tools plugin. It's nice to have.
[JSTImageTools viewNSImage:inWindowNamed:] - JSTImageTools is a class which helps you view your creations. Just pass it an NSImage and a window name, and it'll pop up. In addition to viewNSImage:, there is also viewCIImage: and viewImageBuffer: which takes an JSTOpenCLImageBuffer. You can find samples for both of these in the Image Tools sample directory. However, I'm going to go ahead and show the CIImage sample, since I think it's pretty neat how short it is:
var filter = [JSTQuickCIFilter quickFilterWithKernel:""" kernel vec4 foo(vec4 colorA, vec4 colorB) { vec2 dest = destCoord(); vec4 ret = dest.x < 100.0 ? colorA : colorB; return premultiply(ret); }"""]; [filter addKernelArgument:[CIColor colorWithString:"0.0 0.0 1.0 1.0"]]; [filter addKernelArgument:[CIColor colorWithString:"0.0 1.0 1.0 0.5"]]; var img = [filter outputImage]; [JSTImageTools viewCIImage:img inWindowNamed:"test" extent:CGRectMake(0, 0, 400, 200)];
That code will give you this:
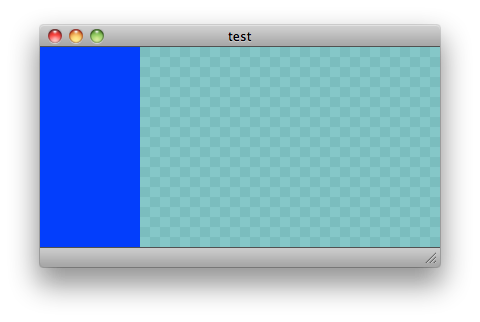
The kernel is nothing earth shattering, but it's super easy to get up and running to test your ideas in matter of seconds. I even ported the Color Wheel generator to it.
So in summary, JSTalk is now a great way to prototype UI controls or do image processing with Core Image or OpenCL. It's almost like Logo, but for Cocoa programmers.